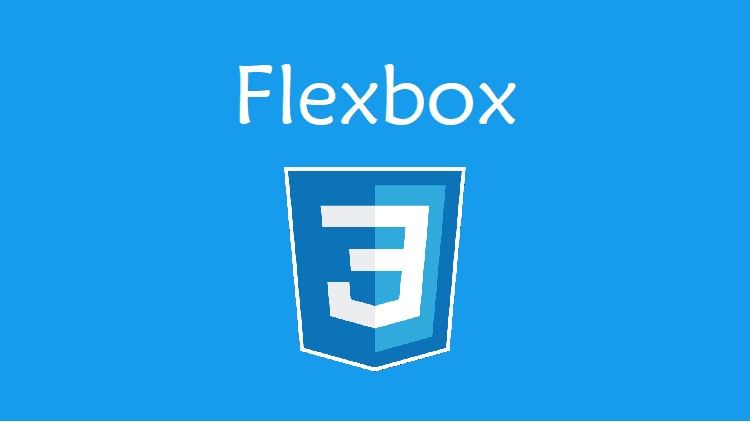
Mastering Flexbox in CSS: A Comprehensive Guide
In the world of modern web design, Flexbox has become an essential tool for creating responsive and flexible layouts. Whether you’re a beginner or an experienced developer, understanding Flexbox is crucial for building dynamic and adaptable web pages. In this guide, we’ll dive into the fundamentals of Flexbox, explore its key properties, and provide practical examples to help you master this powerful layout module.
What is Flexbox?
Flexbox, short for “Flexible Box Layout,” is a CSS layout module designed to provide a more efficient way to distribute space and align items within a container. It simplifies the process of creating complex layouts by allowing elements to adapt and reflow based on the available space.
Basic Concepts
Before we jump into the properties, let’s understand some basic concepts:
- Flex Container: The parent element that holds the flex items. It is defined by setting the
display
property toflex
orinline-flex
. - Flex Items: The child elements within the flex container that are laid out using Flexbox.
Flex Container Properties
1. display: flex
To enable Flexbox, you need to set the display
property of the container to flex
:
.container {
display: flex;
}
2. flex-direction
The flex-direction
property defines the direction in which the flex items are placed within the container:
row
(default): Items are placed in a row, from left to right.row-reverse
: Items are placed in a row, from right to left.column
: Items are placed in a column, from top to bottom.column-reverse
: Items are placed in a column, from bottom to top.
.container {
flex-direction: row;
}
3. justify-content
The justify-content
property aligns the flex items along the main axis (horizontally in a row, vertically in a column):
flex-start
(default): Items are aligned to the start of the container.flex-end
: Items are aligned to the end of the container.center
: Items are centered within the container.space-between
: Items are evenly distributed with equal space between them.space-around
: Items are evenly distributed with equal space around them.
.container {
justify-content: center;
}
4. align-items
The align-items
property aligns the flex items along the cross axis (vertically in a row, horizontally in a column):
stretch
(default): Items stretch to fill the container.flex-start
: Items are aligned to the start of the container.flex-end
: Items are aligned to the end of the container.center
: Items are centered within the container.baseline
: Items are aligned along their baselines.
.container {
align-items: center;
}
5. flex-wrap
The flex-wrap
property controls whether the flex items should wrap or not:
nowrap
(default): Items do not wrap.wrap
: Items wrap onto multiple lines.wrap-reverse
: Items wrap onto multiple lines in reverse order.
.container {
flex-wrap: wrap;
}
Flex Item Properties
1. flex-grow
The flex-grow
property defines the ability of a flex item to grow relative to the rest of the flex items:
.item {
flex-grow: 1;
}
2. flex-shrink
The flex-shrink
property defines the ability of a flex item to shrink relative to the rest of the flex items:
.item {
flex-shrink: 1;
}
3. flex-basis
The flex-basis
property defines the initial size of a flex item before any remaining space is distributed:
.item {
flex-basis: 200px;
}
4. align-self
The align-self
property allows a single item to be aligned differently than the other items:
auto
(default): Inherits the value ofalign-items
from the parent.flex-start
: Aligns the item to the start of the container.flex-end
: Aligns the item to the end of the container.center
: Centers the item within the container.baseline
: Aligns the item along its baseline.stretch
: Stretches the item to fill the container.
.item {
align-self: flex-end;
}
Practical Examples
Example 1: Simple Row Layout
<div class="container">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
</div>
.container {
display: flex;
justify-content: space-between;
}
.item {
background-color: #f0f0f0;
padding: 10px;
margin: 5px;
}
Example 2: Column Layout with Wrapping
<div class="container">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<div class="item">Item 4</div>
</div>
.container {
display: flex;
flex-direction: column;
flex-wrap: wrap;
height: 200px;
}
.item {
background-color: #f0f0f0;
padding: 10px;
margin: 5px;
}
Conclusion
Flexbox is an incredibly powerful tool for creating flexible and responsive layouts in CSS. By understanding and utilizing its properties, you can build complex designs with ease and ensure your web pages look great on any device. Experiment with different properties and combinations to see the full potential of Flexbox in your projects. Happy coding!